Instead of using the hardware timers of the microcontroller, timers can also be emulated in software. Thus, based on a single hardware timer, any number of software timers can be implemented. For the Arduino environment there exist several libraries that provide software timers. One of them is the library arduino-timer.
As an example two light emitting diodes (LED) shall blink controlled by two software timers: The green LED blinks with a frequency of 5Hz (alternating 100ms on and 100ms off). The red LED shall light up once for 2 seconds after a keystroke. I.e. we need an endless running 100ms timer and a 2s timer running only once each.
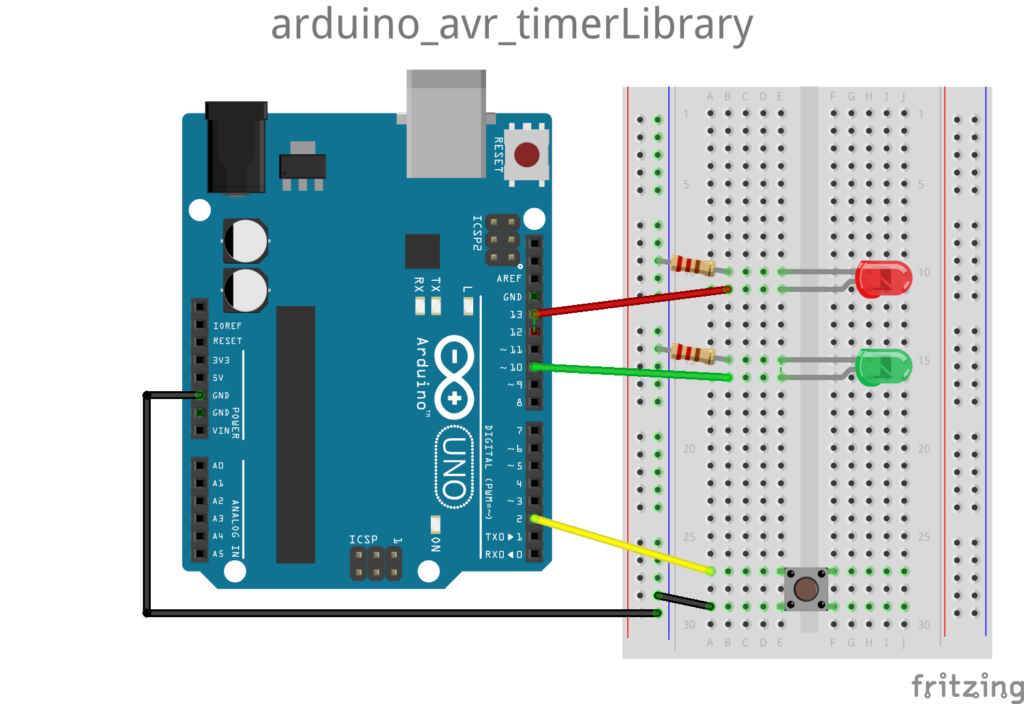
The program / sketch (https://github.com/schaeren/micro_controller/tree/master/arduino_avr/c/arduino_avr_timerLibrary):
#include "Arduino.h" #include "arduino-timer.h" const int greenLedPin = 10; // green LED const int redLedPin = 13; // red LED const int buttonPin = 2; // button // Delay to be used to debounce button [ms] unsigned long debouncingTime = 100; // timer is a 'software timer'. <2> indicates that up to 2 instances may be used concurrently. // One interval timer is used for green LED and one single-shot timer is used for red LED. // For more information see also https://github.com/contrem/arduino-timer Timer<2> timer; unsigned long lastButtonDownTime = 0; bool onSwitchOffRedLed(void *); void onButtonDown() { unsigned int now = millis(); if (now - lastButtonDownTime > debouncingTime) { Serial.print("Button DOWN, last call to onButtonDown() at "); Serial.print(lastButtonDownTime); Serial.print("ms - current time is "); Serial.print(now); Serial.println("ms"); lastButtonDownTime = now; digitalWrite(redLedPin, HIGH); timer.in(2000, onSwitchOffRedLed); } } bool onSwitchOffRedLed(void *) { digitalWrite(redLedPin, LOW); return false; // stop timer } bool onTimer(void *) { digitalWrite(greenLedPin, !digitalRead(greenLedPin)); return true; // keep timer running } void setup() { pinMode(greenLedPin, OUTPUT); pinMode(redLedPin, OUTPUT); pinMode(buttonPin, INPUT_PULLUP); Serial.begin(115200); attachInterrupt(digitalPinToInterrupt(buttonPin), onButtonDown, FALLING); timer.every(100, onTimer); // timer for green led (blicking with 5Hz) } void loop() { timer.tick(); }
Timer<2> timer;
defines a variable which can contain a maximum of 2 cuncurrently running timers.
timer.every(100, onTimer);
starts a timer which calls the function onTimer()
every 100ms. As long as this function returns true
, this timer continues to run.
For the button (buttonPin
) an interrupt handler is registered, which calls the interrupt handler onButtonDonw()
when the button is pressed down (falling edge) (an introduction to external interrupts can be found here). The interrupt handler function debounces the button, lets the LED light up and starts a second timer with timer.in(2000, onSwitchOffRedLed);
which calls the function onSwitchOffRedLed()
once after 2000ms, which then switches the LED off again.
More information about the used library: https://github.com/contrem/arduino-timer